JavaScript Data Structures - Stack
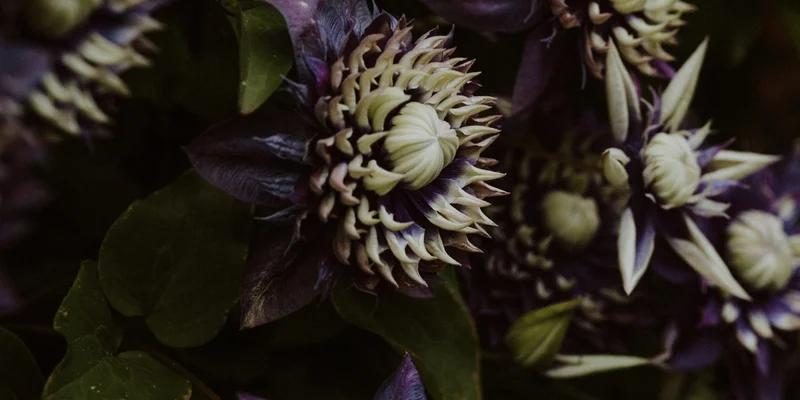
Definition
A stack is a linear data structure that behaves like a real-world stack of items. It follows a last in, first out (LIFO) order of operations, similar to its real-world counterpart. This means that new items are added to the top of the stack and items are removed from the top of the stack as well.
The main operations of a stack data structure are:
push
: Adds an element to the top of the stackpop
: Removes an element from the top of the stackpeek
: Retrieves the element at the top of the stack, without removing itisEmpty
: Checks if the stack is empty
Implementation
class Stack { constructor() { this.items = []; } push(item) { this.items.unshift(item); } pop(item) { return this.items.shift(); } peek(item) { return this.items[0]; } isEmpty() { return this.items.length === 0; } }
- Create a
class
with aconstructor
that initializes an empty array,items
, for each instance. - Define a
push()
method, which usesArray.prototype.unshift()
to add an element to the start of theitems
array. - Define a
pop()
method, which usesArray.prototype.shift()
to remove an element from the start of theitems
array. - Define a
peek()
method, which retrieves the value of the first element in theitems
array, without removing it. - Define an
isEmpty()
method, which usesArray.prototype.length
to determine if theitems
array is empty.
const stack = new Stack(); stack.push('apples'); stack.push('oranges'); stack.push('pears'); stack.isEmpty(); // false stack.peek(); // 'pears' stack.pop(); // 'pears' stack.pop(); // 'oranges' stack.pop(); // 'apples' stack.isEmpty(); // true