JavaScript Data Structures - Queue
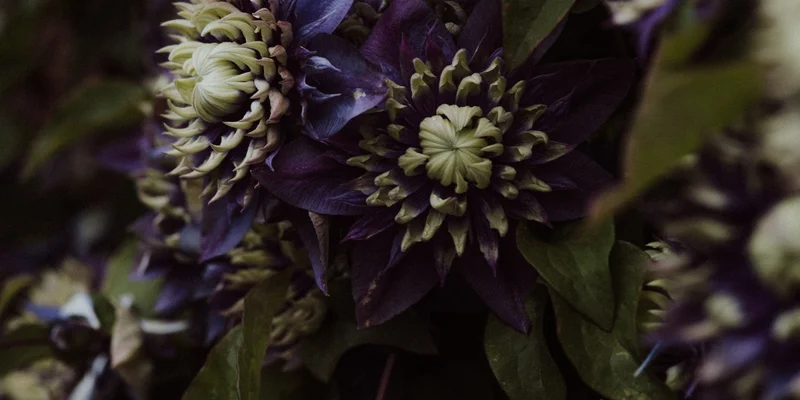
Definition
A queue is a linear data structure that behaves like a real-world queue. It follows a first in, first out (FIFO) order of operations, similar to its real-world counterpart. This means that new items are added to the end of the queue, whereas items are removed from the start of the queue.
The main operations of a queue data structure are:
enqueue
: Adds an element to the end of the queuedequeue
: Removes an element from the start of the queuepeek
: Retrieves the element at the start of the queue, without removing itisEmpty
: Checks if the queue is empty
Implementation
class Queue { constructor() { this.items = []; } enqueue(item) { this.items.push(item); } dequeue() { return this.items.shift(); } peek() { return this.items[0]; } isEmpty() { return this.items.length === 0; } }
- Create a
class
with aconstructor
that initializes an empty array,items
, for each instance. - Define an
enqueue()
method, which usesArray.prototype.push()
to add an element,item
, to the end of theitems
array. - Define a
dequeue()
method, which usesArray.prototype.shift()
to remove an element from the start of theitems
array. - Define a
peek()
method, which retrieves the value of the first element in theitems
array, without removing it. - Define an
isEmpty()
method, which usesArray.prototype.length
to determine if theitems
array is empty.
const queue = new Queue(); queue.isEmpty(); // true queue.enqueue('A'); queue.enqueue('B'); queue.enqueue('C'); queue.enqueue('D'); queue.enqueue('E'); queue.isEmpty(); // false queue.peek(); // 'A' queue.dequeue(); // 'A' queue.dequeue(); // 'B' queue.dequeue(); // 'C'