React useHash hook
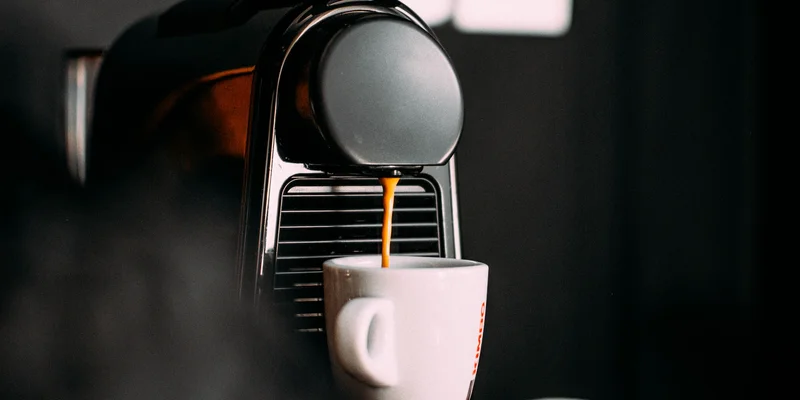
Tracks the browser's location hash value, and allows changing it.
- Use the
useState()
hook to lazily get thehash
property of theLocation
object. - Use the
useCallback()
hook to create a handler that updates the state. - Use the
useEffect()
hook to add a listener for the'hashchange'
event when mounting and clean it up when unmounting. - Use the
useCallback()
hook to create a function that updates thehash
property of theLocation
object with the given value.
const useHash = () => { const [hash, setHash] = React.useState(() => window.location.hash); const hashChangeHandler = React.useCallback(() => { setHash(window.location.hash); }, []); React.useEffect(() => { window.addEventListener('hashchange', hashChangeHandler); return () => { window.removeEventListener('hashchange', hashChangeHandler); }; }, []); const updateHash = React.useCallback( newHash => { if (newHash !== hash) window.location.hash = newHash; }, [hash] ); return [hash, updateHash]; }; const MyApp = () => { const [hash, setHash] = useHash(); React.useEffect(() => { setHash('#list'); }, []); return ( <> <p>window.location.href: {window.location.href}</p> <p>Edit hash: </p> <input value={hash} onChange={e => setHash(e.target.value)} /> </> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <MyApp /> );